Hello everyone,
I struggle with the following problem for a long time now, not knowing how to map (using Hibernate/JPA annotations) this class/dependancy construct correctly, and I really hope you can help me with this.
To simplify things, I created two example classes, representing my mapping problem (please do not question if the classes make sense, these are just only examples to get the point of the problem :). These classes look like this:
Code:
@Entity
public class Plane {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@OneToOne(mappedBy = "plane", cascade = CascadeType.ALL)
private Wing leftWing;
@OneToOne(mappedBy = "plane", cascade = CascadeType.ALL)
private Wing rightWing;
// [...] (Getters/Setters)
}
Code:
@Entity
public class Wing {
public enum WingPosition {
LEFT, RIGHT
}
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@OneToOne
private Plane plane;
@Enumerated(EnumType.STRING)
private WingPosition wingPosition;
private Boolean defect;
// [...] (Getters/Setters)
}
To wire together theses objects, one can do the following:
Code:
Plane plane = new Plane();
Wing leftWing = new Wing();
leftWing.setPlane( plane );
leftWing.setWingPosition( WingPosition.LEFT );
leftWing.setDefect( false );
Wing rightWing = new Wing();
rightWing.setPlane( plane );
rightWing.setWingPosition( WingPosition.RIGHT );
rightWing.setDefect( true );
plane.setLeftWing( leftWing );
plane.setRightWing( rightWing );
When I now persist this with Hibernate, everything is okay, there is one Plane in the Plane-Table and two Wings in the Wing-Table.
But, loading this construct is not possible.
It is not possible, because Hibernate, when loading the Wing for a Plane, exprects exactly one occurrence of a Wing with the ID of the Plane, but there are two Wings referring to the same Plane (ID).
To make the situation clear and to show what I want to achieve, I created this image of the two tables:
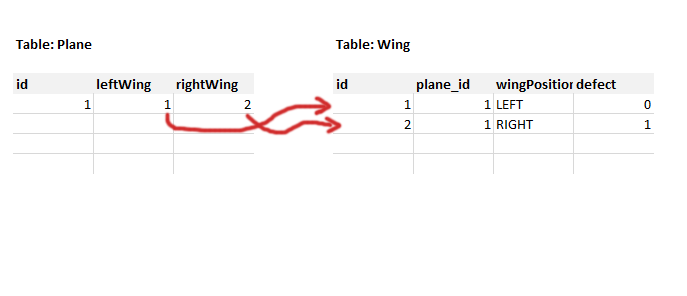
So the simple question is, how can I tell Hibernate to map the Property Plane.leftWing to that Object, which has the Property Wing.WingPosition set to "LEFT", and Plane.rightWing to the Object with Wing.WingPosition set to "RIGHT"?
I was thinking of using a compound key in any way, but I can't figure out how to do that.
(Currently, my workaround is to use a List of Wings, and to do the necessary logic to get the right and left Wing in Java)
I can't imagine why there shoudn't be some easy way to map this correctly.
My Hibernate version: 3.5.6
I really appreciate your help!
Thanks a lot!